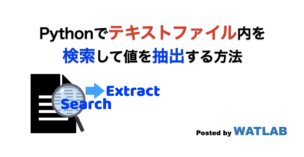
商用ソフトの中にはテキストファイルで設定を用意するものが多くあります。テキストファイルをプログラム的に処理する事で業務自動化が可能です。ここではPythonを使ってテキストファイルの中身を特定のキーワードで検索し、任意の設定値を取得するコード例を紹介します。
こんにちは。wat(@watlablog)です。今回はテキストファイル内のキーワード検索をやってみます!
この記事はメモ的に書いているので、目次から参照したい項目へジャンプしてご活用ください。
例題のテキストファイル
今回は以下のサンプルファイルを使います。
「Pythonでテキストファイルの読み書きをする時のメモ」でファイルの読み書きを学びましたが、今回は検索できるようになる事を目標にちょっと長い文にしています。
このテキストはとあるソフトの設定ファイルを模擬していますが、手入力で作成したため本物のフォーマットと異なる可能性があります(あくまでサンプル)。
CAE屋さんならピンと来るファイル内容かも知れませんが、ここでは「あるルールに則って文字列で記録された設定ファイル」という理解で十分でしょう。
テキスト検索を活用したPythonコード例
キーワードが何行目にあるか検索するコード
文字列完全一致/行内位置指定で検索
ファイル全体から特定のキーワードが何行目にあるのか検索する事を行いますが、まずは完全に位置を指定してしまう事を考えます。
以下のコードはopen_text_line()関数でファイルを行単位で読み込み、search_keyword()関数に読み込んだリスト形式のテキストと検索したいキーワードを与えて、キーワードが何行目にあるかを探すだけのコードです。
検索キーワードの長さを計算し、抽出した行データから文字を取り出して「==」演算子で比較をしています。
以下が結果です。6行目と15行目(0からカウント)にキーワードがある正解を得ました。
文字列完全一致/行内位置不定で検索(.find)
上記コードは位置を完全に指定していましたが、.findメソッドを使えばもっと簡単に書けます。
.find()は文字列の中に検索キーワードが無い場合に-1を返し、ある場合は何文字目からかを示す位置情報が返って来ます。そのため、if文では-1かどうかを見ています。
文字列を一部含むか/行内位置不定で検索(in)
in演算子を使えば文字列が完全一致しなくても、一部でも含めば検索にヒットします。以下のコードは「NODE」を検索。
「*SET_NODE」2つと「*NODE」にヒットしました。
指定した文字列が整数に変換できるかどうかで検索(try/except)
こちらはint型に変換できるかどうかを調べるためのコード。tryとexceptを使ってエラー検出するという方法を使ってみました。
変換できたのは'000'と'1'だけでしたので正解を得ました。intをfloatにすれば浮動小数点型にも対応する事ができます。
応用例:サンプルファイルから座標値を抽出するコード
ここから先は応用例です。応用例なので実際の使用には各自でコードをチューニングする必要がありますが、単なる事例として参考にメモしておきます。
目標
テキストファイル内のとあるid値に対応する座標を抽出する
手順
- ①キーワードを検索する
テキストファイル内に複数ある「*NODE_SET」(nidをグループ化しているキーワード)を検索します。 - ②set id番号を抽出する
「*NODE_SET」キーワードは固有のsid番号で管理されているため、この番号を抽出します。 - ③node id番号を抽出する
「*NODE_SET」にはnid番号がグルーピングされているため、これを全て抽出します。 - ④キーワード数分繰り返す
「*NODE_SET」は複数存在する場合があるため、繰り返します。 - ⑤抽出したnidに対応するnidを「*NODE」から検索する
nidは「*NODE」設定と紐付けられているので、対応を検索します。 - ⑥座標(x, y, z)を抽出する
検索にヒットしたid番号のみ、座標値を抽出します(ここが本来目的)。 - ⑦抽出したnid分繰り返す
- ⑧抽出結果をcsvに保存
図にすると以下です。
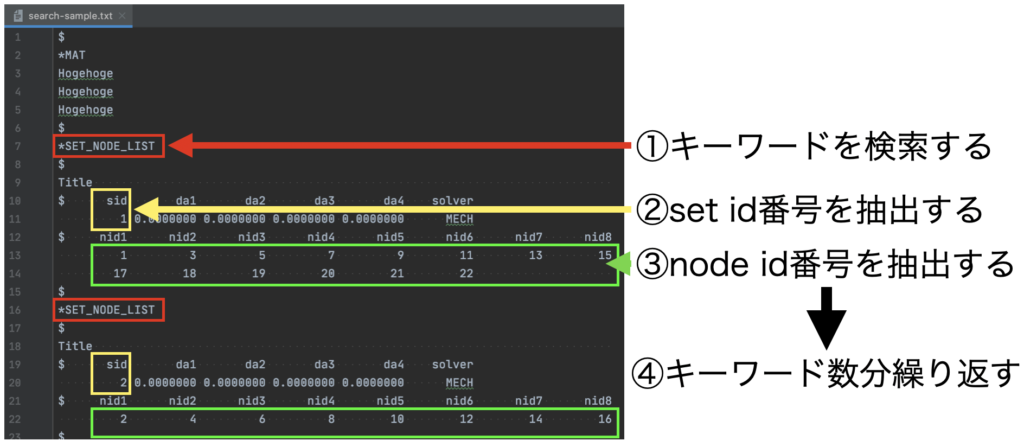
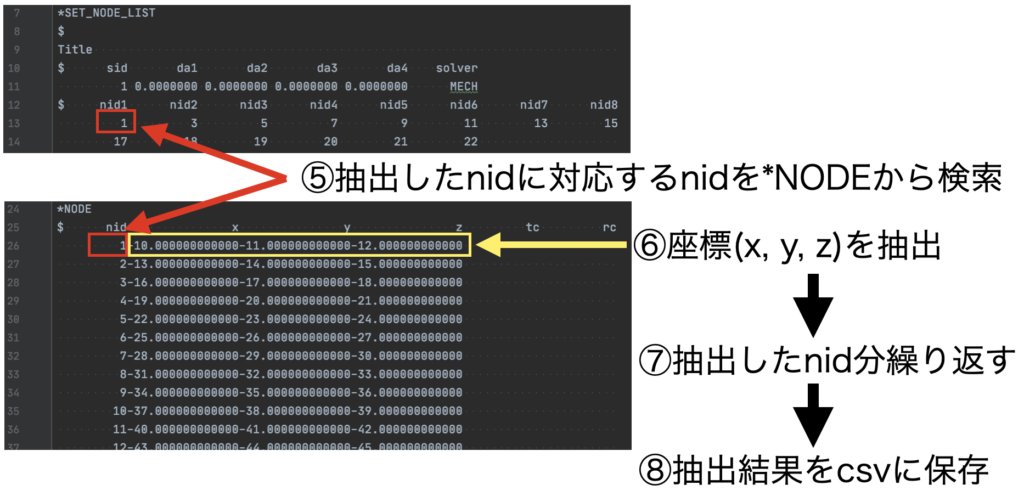
全コード
csvを扱うためPandasをimportしていますが、それ以外はPythonの標準で記述しています。
上で取り扱った基本的な関数を組み合わせたり改造したりして構成しているので、これを読み解いて理解するというよりは、自分が扱いたいテキストファイルでやりたい事を考え、うまくいく方法の参考にする程度で見る方が良い思います。
実行結果
以下が結果です。sid, nid, 座標情報が一つのテーブルにまとまりました。このようにスプレッドシートでまとめる事ができると、その後の処理(この事例では座標変換等)がしやすくなります。
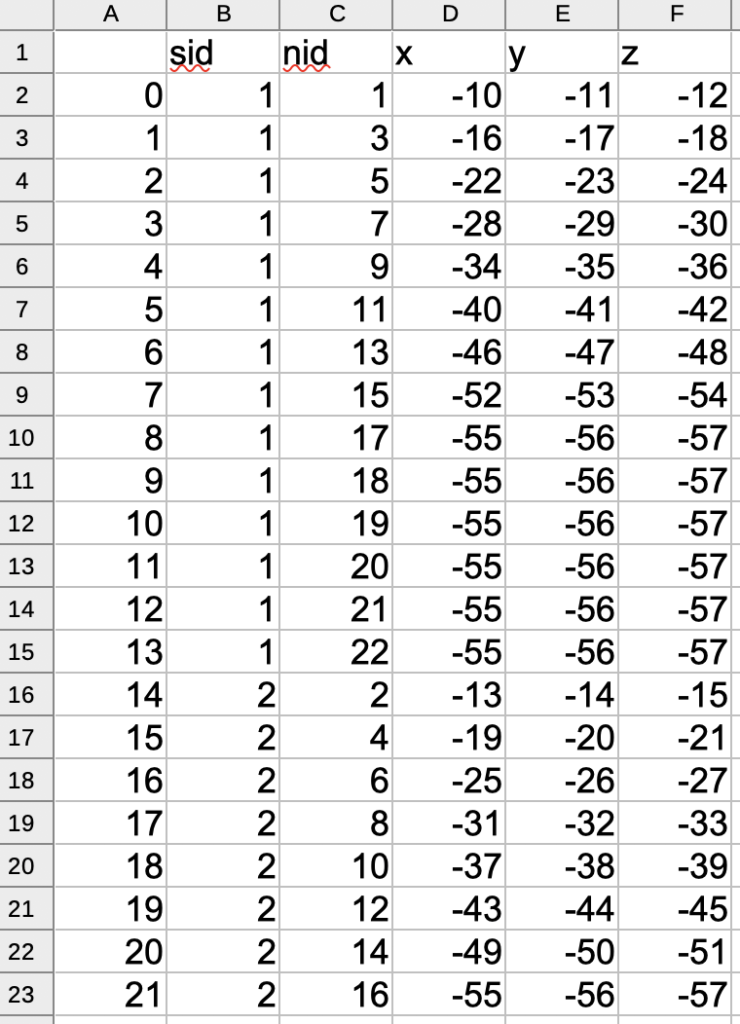
その後の処理もPythonで書く場合は、既にPandasのデータフレームに変換したdfを使う方が効率が良いでしょう。今回のdfには以下のような情報が格納されました。
Pandasは機械学習の前処理、集計といったデータサイエンスが得意なライブラリなので、当ブログではできるだけPandasでテーブルデータを処理しています。
まとめ
本記事はテキストの検索方法として、Pythonで使われている方法やエラー検出の方法を使った例を紹介しました。
また、実際の応用例として、やや複雑なテキストファイルから検索や抽出を使うコードを紹介しました。
なんとなくテキスト検索、抽出の方法がわかってきたと思います!
Twitterでも関連情報をつぶやいているので、wat(@watlablog)のフォローお待ちしています!